Python with Pgeocode and Pandas. Postal codes to geo coordinates.
In some projects, you may need to retrieve geo coordinates from the set of zip codes. pgeocode
is a lightweight Python library for high-performance off-line querying of GPS coordinates, region name and municipality name from postal codes.
You can read this on Medium here.
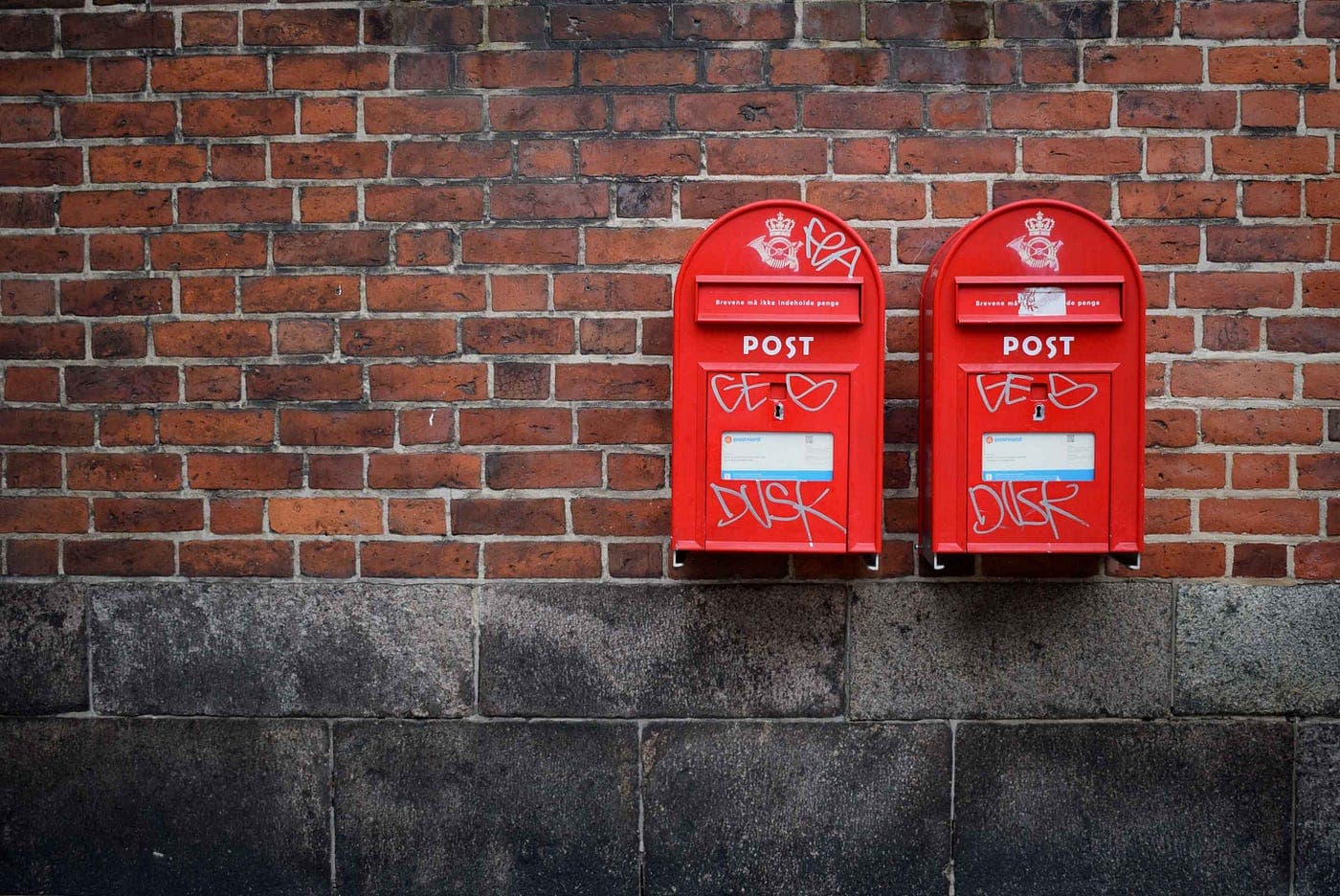
Distances between postal codes and general distance queries are also supported. The database in use includes postal codes for 83 countries.
Check the source here.
Let me quickly show you how to use pgeocode
in one of the real projects where we are tasked to retrieve geo coordinates from the subset of zip codes.
- Let's begin with installing
pgeocode
.
pip install pgeocode
or
conda install -c conda-forge pgeocode
- Then, we import the key libraries for this little project.
import pandas as pd
import os
import pgeocode
- And we import our data to pandas DataFrame to
df
variable
df = pd.read_excel('CH_Post.xlsx')
df
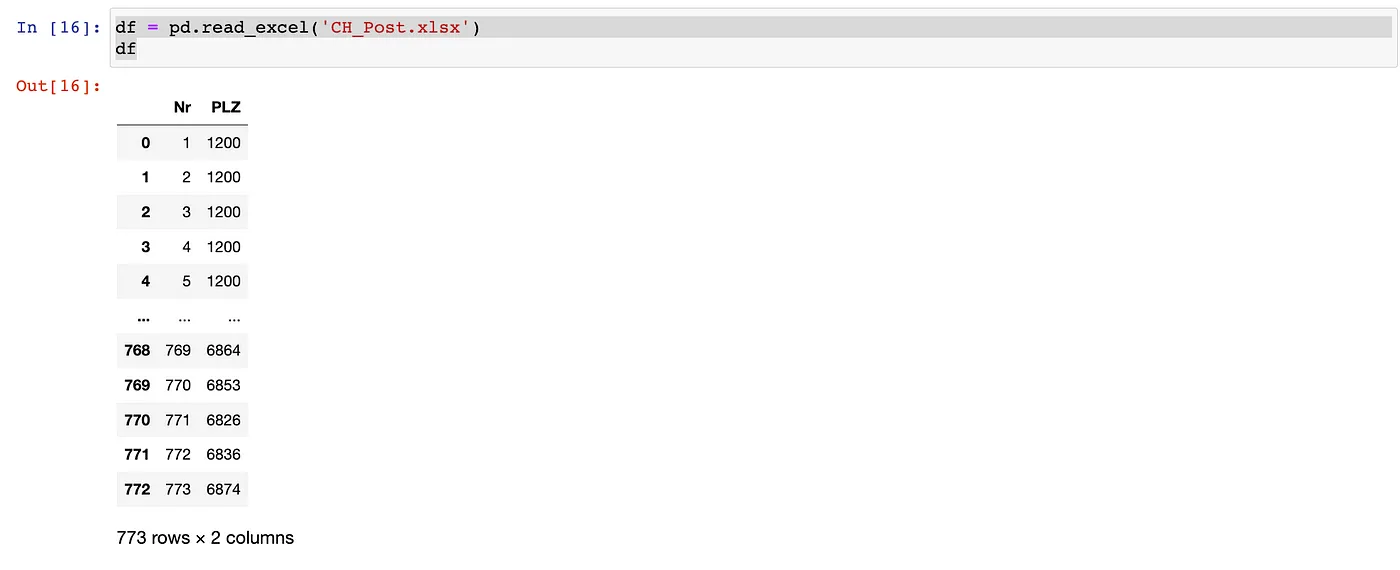
- We are then storing zip codes column to
postal_codes
variable
postal_codes = df["PLZ"].values.astype('str').tolist()
postal_codes
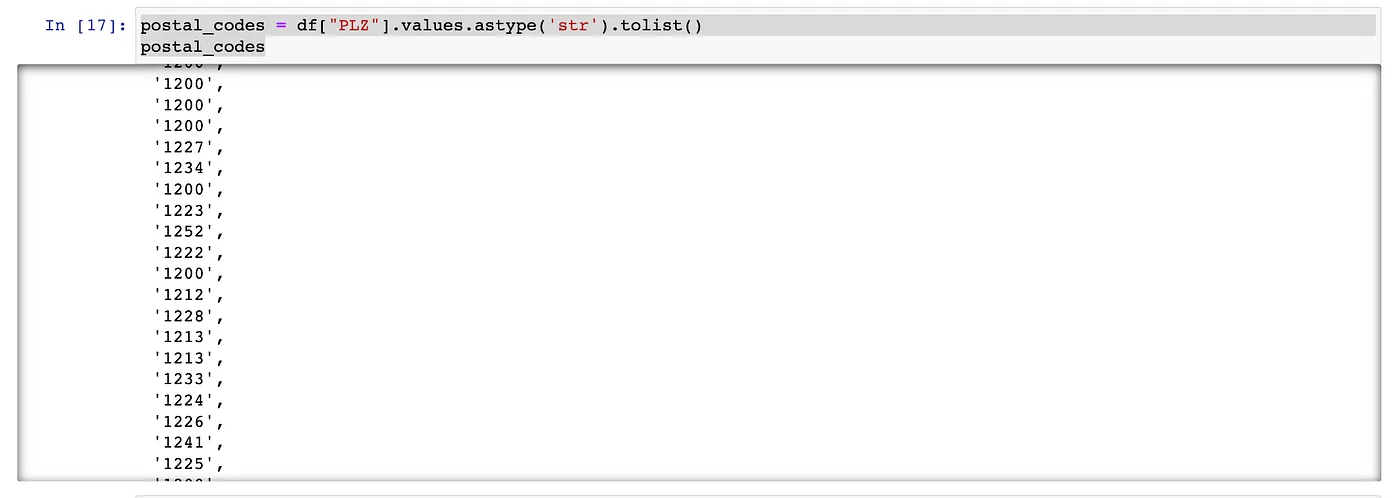
- Subsequently, we initiate our pgeocode library, indicating we are seeking coordinates from the zip codes in Switzerland (providing ISO country code)
nomi = pgeocode.Nominatim('ch')
swiss_post = nomi.query_postal_code(postal_codes)
swiss_post
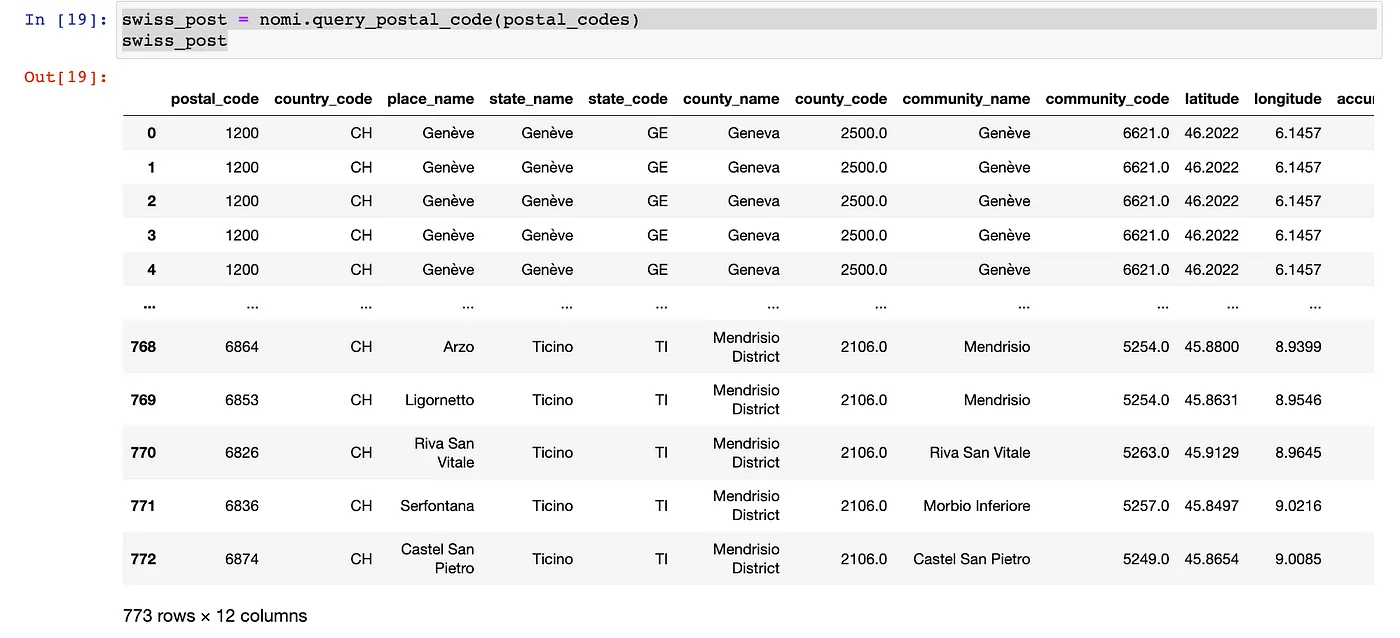
- The last step is to save our newly created dataset to .csv file.
swiss_post.to_csv('CH_Post_with_geocoord.csv')
CONCLUSION
In the six easy steps, you retrieved geo coordinates from the set of zip codes using pandas and pgeocode.